Printing a Modern Experience SharePoint Page – DevScope Ninjas
As Team Manager of the best SharePoint team and forever SharePoint Dev :), I’ve always been looking to develop the next cool feature on top of SharePoint. One of the most-requested cases is printing a SharePoint page OOB, a feature people keep begging for on Office365 User Voice.
Ok then… Challenge Accepted. 🙂
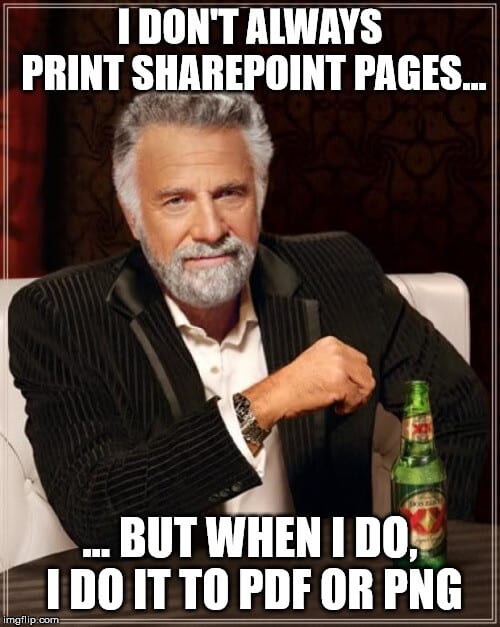
Yes, we did a SharePoint Framework Extension that allows you to export the current page to either PDF or PNG.
Frameworks Recipe
Mixing it all together
Firstly, you should know by now how to develop a SharePoint Framework Extension. If you don’t, the guys at PnP have great samples that let you start exploring it here.
Ok. Now we have our solution ready to develop.
First, we declare my FloatingButton to have 2 buttons. One to export to PDF, and another to export to PNG.
var links = [
{
"bgcolor":"#2980b9",
"icon":"<i class='fa fa-plus'></i>",
},
{
"bgcolor":"#f1c40f",
"color":"fffff",
"icon":"<i class='fa fa-file-pdf-o'></i>",
"id": "printPDF"
},
{
"bgcolor":"#FF7F50",
"color":"fffff",
"icon":"<i class='fa fa-picture-o'></i>",
"id": "printImage"
}
];
var options = {
rotate: true
};
($('#wrapper')as any).jqueryFab(links, options);
Now, if you decide to create a simple PNG from the page, you should know that in the Modern Experience there is a div that only displays the content of the canvas called spPageCanvasContent.
With that in mind, you can now use html2canvas to convert the page’s HTML to canvas and then to a base64 dataUrl. After that, you just need to open this image in a new URL.
var c:any = document.getElementById('spPageCanvasContent');
html2canvas(c,
{
useCORS: true,
}
).
then((canvas) => {
canvasToImg(canvas, imageToPdf, imageLoadError);
});
var canvasToImg = function(canvas, loaded, error){
var dataURL = canvas.toDataURL('image/png'),
img = new Image();
if(!isPdf){
var win = window.open();
win.document.write('<iframe src="' + dataURL + '" frameborder="0" style="border:0; top:0px; left:0px; bottom:0px; right:0px; width:100%; height:100%;" allowfullscreen></iframe>');
}
}
This should be the result for a PNG.

But…if you want to export it to PDF, this can be a little bit trickier. Now that you have the PNG, what you must do is to “paste” it on a PDF page, but keep in mind the size of the image so it doesn’t get distorted.
var imageToPdf = function(){
var pageOrientation = this.width >= this.height ? 'landscape' : 'portrait';
var img = this,
pdf = new jsPDF({
orientation: pageOrientation,
unit: 'px',
format: [img.width, img.height]
}),
pdfInternals = pdf.internal,
pdfPageSize = pdfInternals.pageSize,
pdfScaleFactor = pdfInternals.scaleFactor,
pdfPageWidth = pdfPageSize.width ,
pdfPageHeight = pdfPageSize.height ,
pdfPageWidthPx = pdfPageWidth * pdfScaleFactor,
pdfPageHeightPx = pdfPageHeight * pdfScaleFactor,
imgScaleFactor = Math.min(pdfPageWidthPx / img.width, 1),
imgScaledHeight = img.height * imgScaleFactor,
shiftAmt = 0,
done = false;
var newCanvas = canvasShiftImg(img, shiftAmt, imgScaleFactor, pdfPageHeightPx, pdfPageWidthPx);
pdf.addImage(newCanvas, 'png', 0, 0, pdfPageWidth, 0, null, 'SLOW');
pdf.save('printThisPage.pdf');
};

Hey, but SharePoint does content lazy loading…
Yep, that’s right. So, we did a trick! Probably not the fanciest way to do it, but it works! We just scroll the div down until everything is loaded.
var scrollRegion = $("div[class^='scrollRegion']");
scrollRegion.animate({ scrollTop: scrollRegion.prop("scrollHeight")}, 2000);
What about Responsive?
Yeah… it works as well!
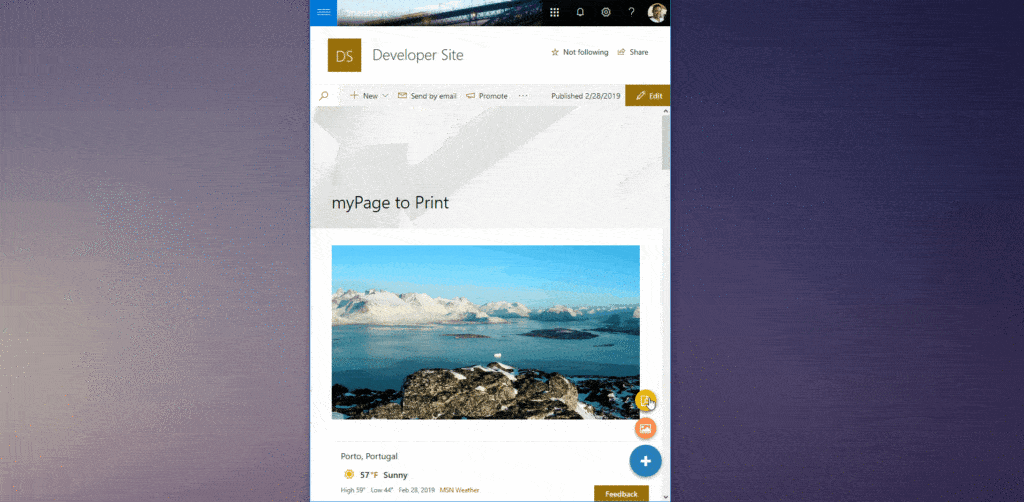
Conclusion
With SPFx there are a lot of new tools and technologies that we can use for SharePoint development, creating great and modern user experiences.
We don’t always need to start from scratch.
Please find the code and the installation package on DevScopeNinjas’ GitHub.
If you have any comments or suggestions leave them in the comments box below!
Sharing is caring!
Reference
Ribeiro, R. (2019). SharePoint Online Multi-language Modern Sites – real case, pros, and cons. Available at: https://devscopeninjas.azurewebsites.net/2019/02/28/printing-modern-experience-sharepoint-page/ [Accessed: 3rd September 2019].